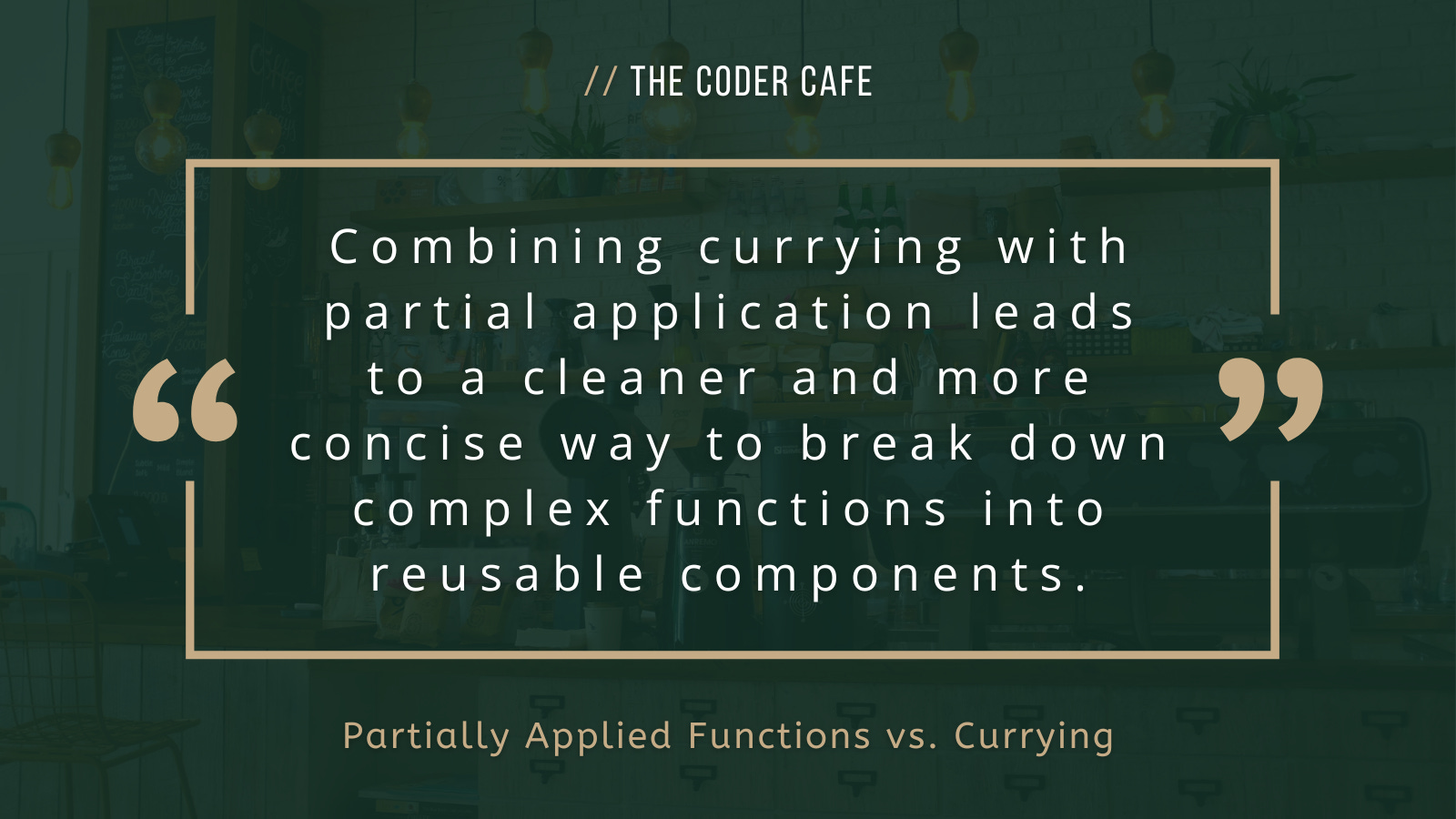
Partially Applied Functions vs. Currying
Combining currying with partial application leads to a cleaner and more concise way to break down complex functions into reusable comp…
Publishes on February 6th, 9:00am. Subscribe now and receive the post in your inbox when it’s live
Available in 18 hours, 53 minutes, and 19 seconds